Introduction
Axios is an HTTP client which makes API requests easier than ever before for developers working with ReactJS. The best thing about Axios is that because of its promise-based design, errors no longer halt execution of code in React, which means the user is not blocked from accessing your app while you deal with problems.
In this article we’ll explore everything you need to know about how to use Axios to make API requests.
What is Axios?
Axios is a promise-based HTTP client that can be used in both browser and Node.js environments, which makes it perfect for apps that are made on top of React. Axios follows the very simple API design pattern, which means you just need to use a function to make a request and use another function to handle the response from the server.
Why is Axios important for us to use?
React and Axios work hand in hand with one another. There is a good chance you will be using React to develop your app, which means you need some form of API communication to store or retrieve data from the server. While there are other options out there such as jQuery, they can become confusing very quickly and don’t offer enough type safety to use in a complex environment like React.
In React, it is handling everything in its own virtual DOM. When we pass data into components via properties, it is actually just adding an object to the component’s state. This means that every component has its own copy of this state and can do what it wants with it. This becomes very useful when you want to update parts of the UI based on the value of a particular property.
How to install Axios in React?
In order to use Axios in React, we have to install it. Go to your project’s root directory and open up the command line that you use to run your npm commands on.
Using NPM, we can install Axios by running the command shown below:
npm install axios --save
Once that is done, we need to import it into our React project.
We are going to useEffect and useState. useEffect is similar to componentDidMount and useState is basically setting the state.
If want more information on useEffect and useState, there are links that you can look at:
Import Code:
import React, { useEffect, useState } from "react";
import axios from "axios";
import './App.css';
The URL we are going to use for this example is a list of USA States with population and latest year population updated.
The first thing we need to do is set the State to save the data from the URL
Since the data from the URL is an object array, we set an empty array in our state
State Code:
import React, { useEffect, useState } from "react";
import axios from "axios";
import './App.css';
export default function App() {
const [data, setData] = useState([]);
}
The next thing we do is set up our useEffect to put our Axois, so we load up our data right away.
import React, { useEffect, useState } from "react";
import axios from "axios";
import './App.css';
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
}, []);
}
Since we are going to get data from the URL, we have to use the GET route. What we need to add is our Axios function and use the “then” arrow function, which is a promise that returns a callback then will return the success or error data that we get. Also in the “then” arrow function, we set the URL data to our state.
import React, { useEffect, useState } from "react";
import axios from "axios";
import './App.css';
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
axios.get("https://datausa.io/api/data?drilldowns=State&measures=Population&year=latest").then((response) => {
setData(response.data.data)
});
}, []);
}
Now we have the data, we are going to print out the state with their latest population number with the year that it was last updated to.
Since it’s an object array, we have to use the map function in our return to print out state, year and population . To add a comma for the numbers, I added toLocaleString to the population for each state
Full Code:
import React, { useEffect, useState } from "react";
import axios from "axios";
import './App.css';
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
axios.get("https://datausa.io/api/data?drilldowns=State&measures=Population&year=latest").then((response) => {
setData(response.data.data)
});
}, []);
return(
{data.map(info =>
State: {info.State}, Latest Year: {info.Year}, Population: {info.Population.toLocaleString("en-US")}
)}
)
}
Here is the result:
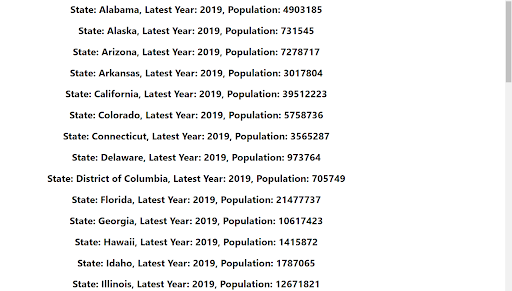
There are more states, this is just a sample of the result. Once you finish the result, it will show you all of the states with it’s latest year update and population
Conclusion
Axios can be used to communicate with an API server and retrieve data from it, which we can use in our React app. Once you get the hang of how Axios works and what problems it solves, you will find yourself reaching for it every time you need to handle your app’s HTTP requests