Introduction
JavaScript has changed the way we develop web pages and mobile applications. With frameworks like React, it’s possible to write components in a “functional” style that is easy to understand, test, and reuse.
In this tutorial, I’ll show you how to filter an array in React!
What is the filtering function in React?
This function pretty much explains itself. It filters out the array based on what you want. How the process works is that it loops for each index of the array and its value. It checks if the value is included or excluded based on the condition. This is similar to adding a condition in a for loop.
The thing is that we are actually not filtering, filter is a Javascript function that does the job for us on an array type object.
Here are different examples of filtering that could be helpful for you when are doing React
Example 1: Filtering Array With String
In this example, we are filtering an array that contains all string
Our sample array will be a list of colours.
const colors = ['Blue', 'Green' , 'Red', 'Black', 'Orange'];
Here is the full code for filtering string
const colors = ['Blue', 'Green' , 'Red', 'Black', 'Orange'];
return(
{colors.filter(color => color.toUpperCase().includes('R')).map(filteredColor => (
{filteredColor}
))}
The first thing we did was put the array that we wanted. In the div that we created, put open up a curly bracket. After that, we put the array name that we want to do the filter on. To perform what filter or condition you want for your array, open a bracket. Put in a name that you want to call each item, we put it as “colour”.
Put an arrow function, then you can do your condition. Since we want it to be a case incentive filter, we made it uppercase, so it would be easier to tell which value has a specific letter. To get the value that has the specific letter put includes, with the letter that we want.
Then put the map function and then print out filtered values
Here is our result:
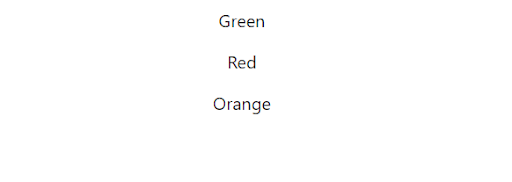
Full Code including imports:
import React from "react";
import './App.css';
export default function App() {
const colors = ['Blue', 'Green' , 'Red', 'Black', 'Orange'];
return(
{colors.filter(color => color.toUpperCase().includes('R')).map(filteredColor => (
{filteredColor}
))}
)
}
Example 2: Filtering Array with Numbers
This example is similar to the first example, but this time with numbers instead of string values.
Sample array of numbers
const numbers = [312, 32, 31, 453, 100, 49, 1, 235, 91, 3];
Here is the full code for filtering numbers
const numbers = [312, 32, 31, 453, 100, 49, 1, 235, 91, 3];
return(
{numbers.filter(number => number > 50).map(filteredNumber => (
{filteredNumber}
))}
)
Similar to the first example, we put the name of our array that we want to filter. Then we put the condition that we want, which is any number greater than 50
After we put the map function, we print out the result
Here is our result:
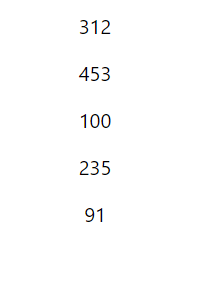
Full Code including imports:
import React from "react";
import './App.css';
export default function App() {
const numbers = [312, 32, 31, 453, 100, 49, 1, 235, 91, 3];
return(
{numbers.filter(number => number > 50).map(filteredNumber => (
{filteredNumber}
))}
)
}
Example 3: Using An Array Object To Filter Out
Now instead of a regular array, we are going to use an array of objects. It is similar to a regular array, but just with an extra step
Our array has expanded into an array of objects for this example. Here is how our array of objects is going to look like
const Occupation = [
{
name: 'Bob',
job: 'Dentist'
},
{
name: 'Micheal',
job: 'Banker'
},
{
name: 'James',
job: 'Banker'
},
{
name: 'Kyle',
job: 'Doctor'
},
{
name: 'Larry',
job: 'Janitor'
}
]
Here is the full code
const Occupation = [
{
name: 'Bob',
job: 'Dentist'
},
{
name: 'Micheal',
job: 'Banker'
},
{
name: 'James',
job: 'Banker'
},
{
name: 'Kyle',
job: 'Doctor'
},
{
name: 'Larry',
job: 'Janitor'
}
]
return(
{Occupation.filter(person => person.job.toUpperCase().includes("O")).map(filteredPerson => (
{filteredPerson.name}'s job is {filteredPerson.job}
))}
)
Similar to the first and second examples, we put the filter on that array that we want, except in the conditioning part we have to choose the condition that we want. Since it’s an object of arrays. If we want to filter the job key, what we do is put “person.job” instead of the person array.
After we put the map function, when you print out the result, we print the array with the key that we want.
Here is final result:
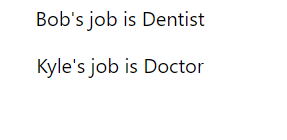
Full Code including imports:
import React from "react";
import './App.css';
export default function App() {
const Occupation = [
{
name: 'Bob',
job: 'Dentist'
},
{
name: 'Micheal',
job: 'Banker'
},
{
name: 'James',
job: 'Banker'
},
{
name: 'Kyle',
job: 'Doctor'
},
{
name: 'Larry',
job: 'Janitor'
}
]
return(
{Occupation.filter(person => person.job.toUpperCase().includes("O")).map(filteredPerson => (
{filteredPerson.name}'s job is {filteredPerson.job}
))}
)
}
Conclusion
We hope that this tutorial helps you understand more how filtering works in React. Or if you’ve already known about it, then at least it would help you refresh your memory on the basics!